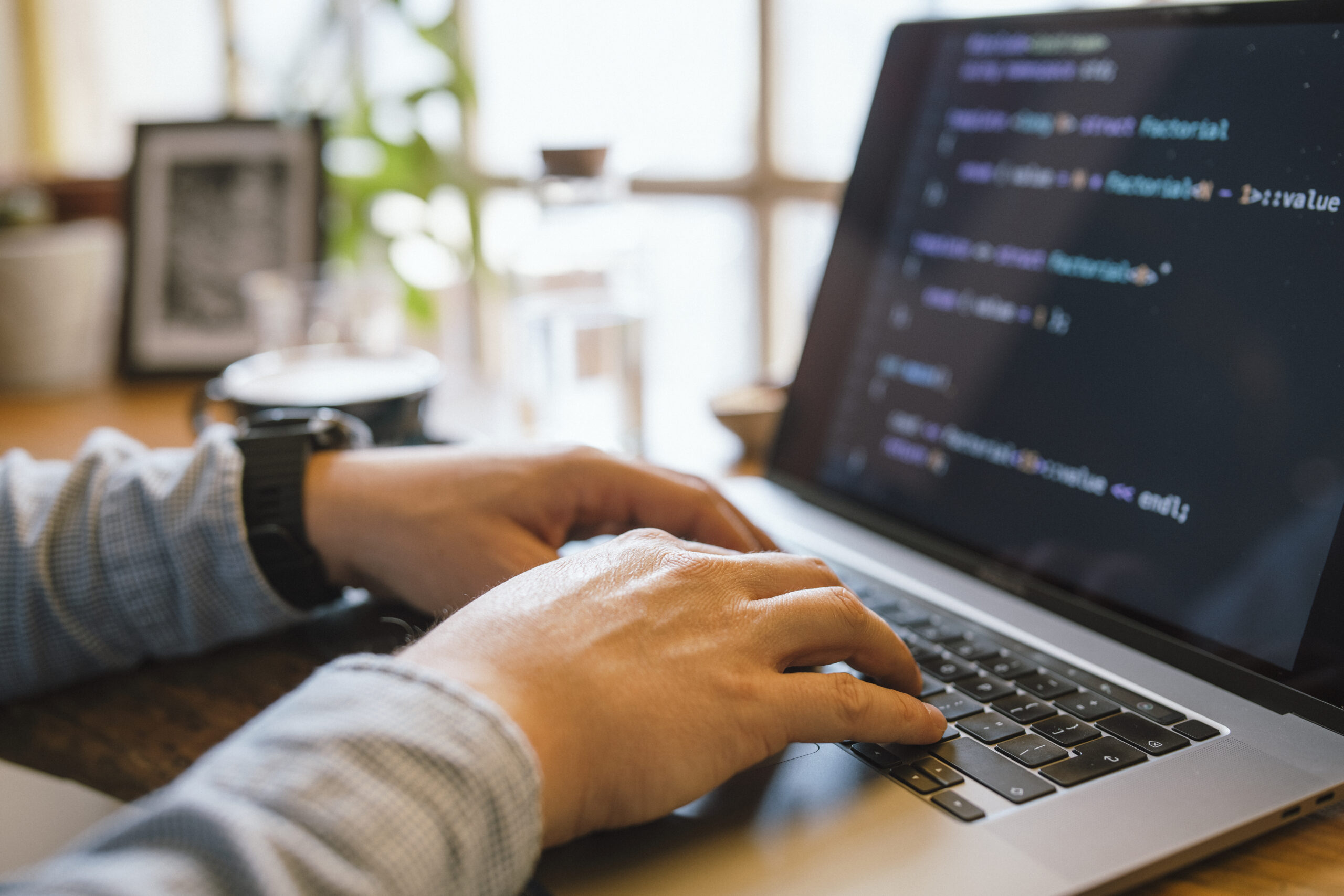
Debugging is The most necessary — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of stress and substantially boost your productiveness. Listed below are a number of methods to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the applications they use everyday. When composing code is 1 part of enhancement, figuring out the best way to interact with it correctly through execution is equally important. Modern-day advancement environments come Outfitted with effective debugging abilities — but many builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step as a result of code line by line, and also modify code on the fly. When applied properly, they Permit you to observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They assist you to inspect the DOM, check community requests, see true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Command methods like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic modifications.
In the end, mastering your applications usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Just before jumping into your code or building guesses, developers require to create a reliable natural environment or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances less than which the bug occurs.
As you’ve gathered sufficient facts, attempt to recreate the condition in your local natural environment. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions Later on.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources much more efficiently, examination prospective fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint right into a concrete obstacle — Which’s the place builders prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where it took place, and often even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Quite a few developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the accurate root induce. Don’t just copy and paste mistake messages into serps — go through and have an understanding of them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line variety? What module or function activated it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Check associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common events (like successful get started-ups), Alert for prospective problems that don’t crack the applying, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant determination points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging technique, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To properly detect and fix bugs, developers need to tactic the procedure similar to a detective solving a mystery. This state of mind aids stop working intricate challenges into workable parts and adhere to clues logically to uncover the root result in.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you could without the need of leaping to conclusions. Use logs, exam scenarios, and person stories to piece collectively a clear image of what’s happening.
Next, form hypotheses. Talk to your self: What might be creating this behavior? Have any variations not long ago been manufactured on the codebase? Has this concern occurred right before less than related conditions? The objective is to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Try and recreate the trouble inside a managed setting. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay shut focus to small facts. Bugs usually disguise from the minimum envisioned areas—similar to a missing semicolon, an off-by-one error, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may well hide the true issue, just for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and develop into more practical at uncovering hidden troubles in elaborate systems.
Compose Assessments
Producing checks is among the most effective approaches to increase Gustavo Woltmann AI your debugging competencies and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that offers you self-confidence when producing adjustments to the codebase. A very well-tested application is easier to debug because it enables you to pinpoint precisely in which and when a difficulty happens.
Start with unit tests, which focus on individual functions or modules. These compact, isolated checks can immediately expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glance, appreciably minimizing time invested debugging. Device assessments are In particular valuable for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Up coming, combine integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your software function alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to think critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge instances. This standard of knowing The natural way prospects to higher code structure and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is just stepping away. Getting breaks can help you reset your intellect, cut down frustration, and infrequently see The difficulty from the new standpoint.
If you're far too near to the code for way too very long, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. In this point out, your Mind will become a lot less productive at difficulty-solving. A short wander, a espresso break, or even switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power plus a clearer state of mind. You might quickly discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, however it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your brain House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you a little something beneficial should you make time to replicate and review what went wrong.
Start by asking oneself several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it are actually caught before with better practices like device tests, code assessments, or logging? The responses normally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially potent. Whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, some of the ideal developers will not be the ones who publish perfect code, but individuals who continuously find out from their issues.
Ultimately, Each individual bug you repair provides a new layer to the talent set. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a far more efficient, assured, and able developer. Another time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.